Core
The very heart of the Remoting SDK is based on Channels, Messages, Envelopes and Services. Two more components used under the hood are Interfaces and Invokers.
Don't be afraid of these words, things are actually simpler than they look. Most steps of the data exchange process are hidden from the developer. Of course they can be accessed and fine-tuned if needed yet most scenarios don't require developer to do that.
Let's take a look at the 'Client calls the server' scenario:
var methodCallResult = serviceProxy.DoSomething();
Looks simple, doesn't it?
Here's what happens from the Remoting SDK's point of view:
The client code calls method of some object serviceProxy. Here, serviceProxy is an instance of the auto-generated proxy class, so calling the serviceProxy.DoSomething() method actually means the following:
-
Sending out a request. The Interface class serializes the request to the server using the configured Message. The Message is then wrapped by the attached Envelopes, if any are set up (f.e. data is encrypted somehow). Then the resulting data package is sent over the wire by the Client Channel.
-
Processing the request on the server side. The Server Channel retrieves the data package and pushes it to the Message component. Message unwraps it using the attached Envelopes, if needed. The resulting data package is provided to the Invoker, which reads the requested service, method names and method parameters from it. Then the Service method is invoked, which does the actual job. After the service method has finished, its result (or any exceptions occurred) are serialized, wrapped, and sent back over the wire using the Server Channel (yes, the processing pipeline is similar to step 1).
-
Retrieving the response. The Client Channel retrieves the data package, unwraps it and provides the serialized data package to the Interface's method that initiated the remote service call. This method then deserializes the provided data package and provides the method result back to the user code.
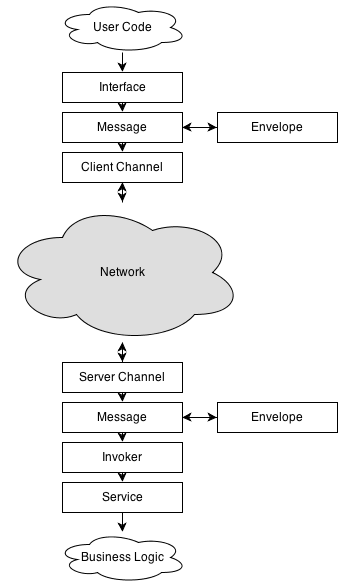
The magic is that the entire process is transparent for the user code on both ends of the wire and doesn't require much attention from the developer. While the service method call pipeline might seem complicated, its performance overhead is negligible (especially for the binary serialization-based Message), making the actual network speed the main issue to care about, not the inner Remoting SDK processes.