MegaDemo sample (JavaScript)
The MegaDemo JavaScript Client is a sample application that works with the MegaDemo Sample (Delphi) or MegaDemo Sample (.NET) server. It demonstrates most of the Remoting SDK for JavaScript features.
Getting started
To start, run the MegaDemo (Delphi) or MegaDemo (.NET) server application and activate any HTTP server. The server is accessed by opening http://localhost:8099/js/ in the web browser (localhost can be changed to the real server address).
Alternatively, you can open \RemObjects Samples\RemObjects SDK for Delphi\Mega Demo\MegaClient JS\MegaDemoJSClient.html
from the disk, enter the server URL in the input box and hit the connect button.
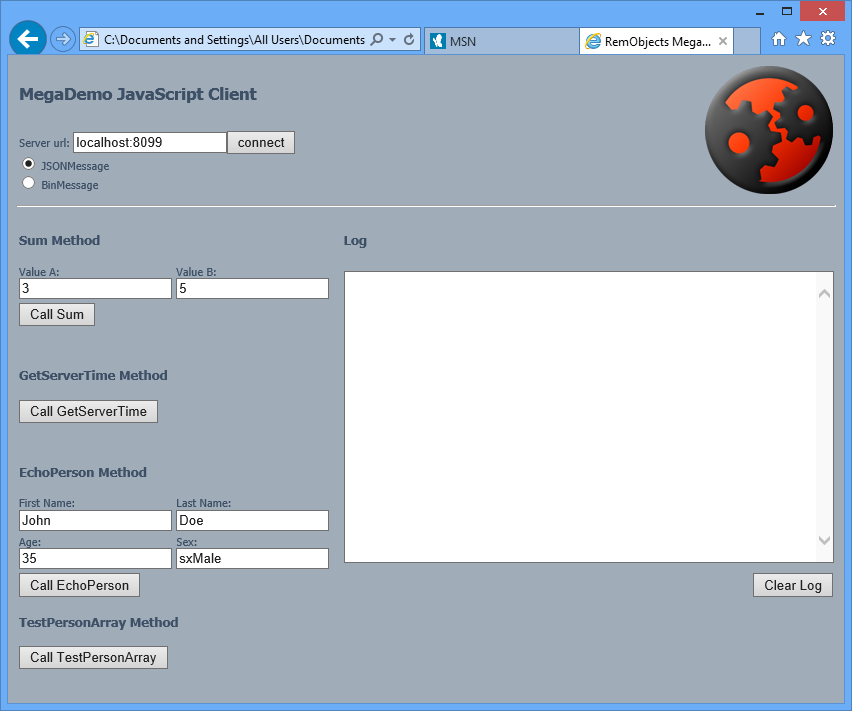
Features overview
Message types
At the moment, RemObjects SDK for JavaScript supports BinMessage and JSONMessage. From the client's perspective, there is no difference between the message types. But in general, Bin vs. JSON means slower but less traffic vs. faster but more traffic.
There is a radio button to switch between message types. When switching, it creates a new message instance and a new channel instance with a corresponding URL (http://localhost:8099/bin or http://localhost:8099/json).
General data types
Illustrated by two simple service methods. Sum
takes two numbers and returns a sum and GetServerTime
just returns the current date/time.
Complex types
EchoPerson
sends a struct to the server and gets it back. TestPersonArray
does the same for an array of structs.
See Using complex types (JavaScript) for more information.
Application internals
Preparation phase
First of all, include the required libraries.
<script type="text/javascript" src="RemObjectsSDK.js"></script>
<script type="text/javascript" src="MegaDemoLibrary_intf.js"></script>
MegaDemoLibrary_intf.js
is generated from the RODL by the JavaScript codegen and contains data types declarations and proxy classes for services, exposed by the server.
Then, before actually calling the server, the following objects are created:
- channel
- message
- service proxy
As usually, in a one-liner:
Service = new MegaDemoService(new RemObjects.SDK.HTTPClientChannel(svcUrl), new RemObjects.SDK.JSONMessage());
Calling the server
When everything is ready, we can call the server method by calling the corresponding service proxy method. It takes input parameters and two callback functions (onSuccess and onError) as its arguments.
function BeginSumMethod() {
AddLineToLog('----------------------');
var A = document.getElementById("editA").value;
var B = document.getElementById("editB").value;
AddLineToLog('Method: Sum; Params: A=' + A + '; B=' + B + ';');
AddToLog('Sending request...');
Service.Sum(A, B, SuccessSumMethod, ErrorSumMethod);
AddLineToLog(' ok.');
AddToLog('Waiting for response... ');
function SuccessSumMethod(result) {
AddLineToLog(' ok.');
AddLineToLog('Result is ' + result);
AddLineToLog('Done.');
alert('Result is ' + result);
}
function ErrorSumMethod(msg, ex) {
if (ex) {
AddLineToLog(" Error: " + ex);
}
else
AddLineToLog(msg.getErrorMessage());
}
}