Modular Server sample (.NET)
Concepts Covered
This sample demonstrates how to create and use the Remoting SDK modular server that allows to load a service module (that is a separate .NET assembly) at runtime which can then be used by the client.
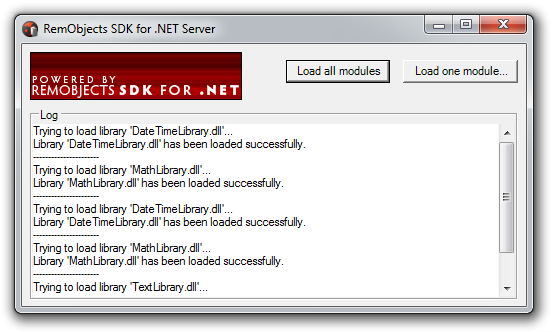
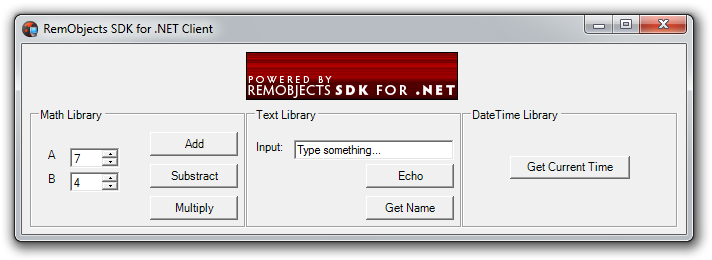
Getting Started
Build and launch the service libraries that are shipped with the solution (DateTimeLibrary, MathLibrary, TextLibrary). The corresponding .dll files will be created. Build and launch the ModularServer server. Click the button Load all modules
to load all available modules or click the button Load one module...
to choose and load a single module. Once loaded, the module(s) will be available to be used by the client. Build and launch the ModularServer client. Choose the library you want to use (Math Library, Text Library, DateTime Library) and click the corresponding button to call the service method. If the client tries to call a method from a module that has not been loaded to the server, the error message Service not found
will be shown.
Examine the code
The ModularServer solution contains the following parts: the server application, the client application and the service libraries.
The service libraries include the full functionality of the service: the RODL file and the corresponding _Intf, _Invk and _Impl files. Each library may be included to the server as a separate service.
The server side represents a Remoting SDK server that allows to load the service libraries using the following code:
// Main.cs file
private void LoadLibrary(string fileName)
{
. . .
try
{
Assembly.LoadFile(fileName);
. . .
}
catch(Exception ex)
{
. . .
}
}
After loading, the service is available to be used by the client.
The client side represents a Remoting SDK client that includes proxy classes (_Intf
files) for all modular services that are shipped with the solution. This means that if you want to add a custom modular service to the server, you need to add the corresponding _Intf
file to the client to work with the service. The client side uses a standard mechanism to work with modular services; it is not different from any other remote service call:
//Using Math library
private void btnAdd_Click(object sender, System.EventArgs e)
{
try
{
MathLibrary.IBasicMathService mathBasicService = MathLibrary.CoBasicMathService.Create(message, clientChannel);
MessageBox.Show(mathBasicService.Add(Int32.Parse(txtOp1.Text), Int32.Parse(txtOp2.Text)).ToString());
}
catch (Exception ex)
{
MessageBox.Show("Exception occured: " + ex.Message);
}
}
//Using Text Library
private void btnEcho_Click(object sender, System.EventArgs e)
{
try
{
TextLibrary.IBasicTextService textBasicService = TextLibrary.CoBasicTextService.Create(message, clientChannel);
MessageBox.Show(textBasicService.Echo(txtText.Text));
}
catch (Exception ex)
{
MessageBox.Show("Exception occured: " + ex.Message);
}
}