Threads sample (.NET)
Concepts Covered
This sample demonstrates how to use RO SDK client components in multithreaded applications.
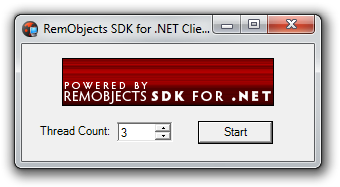
Getting Started
Build and launch the Thread Sample Server. It contains one plain operation that puts the service to sleep for the requested delay and then returns a finish message. Build and launch the Thread Sample Client. Select the thread count you want and click Start
. The client will create the requested number of threads. Each thread will call the service operation one time with a random delay and show the finish message from the server.
Examine the code
The server side represents the Remoting SDK service with the plain operation DoSomethingLong
:
// ThreadServerService_Impl.cs
public class ThreadServerService : RemObjects.SDK.Server.Service, IThreadServerService {
public ThreadServerService() :
base() {
}
public virtual string DoSomethingLong(int Delay) {
Thread.Sleep(Delay);
return "Finished with the long operation. This thread took "+Delay.ToString()+"ms";
}
}
The client side shows how multiple calls can be executed at the same time using multiple threads. When you click the Start
button, the requested number of threads are created:
// Main.cs
private void bStart_Click(object sender, System.EventArgs e)
{
int i = 0;
// private System.Windows.Forms.NumericUpDown nudThreadCount;
for (i=1; i<=nudThreadCount.Value; i++)
{
new Thread(new ThreadStart(ThreadRequest)).Start();
}
}
Each thread creates its own Remoting SDK service, calls the DoSomethingLong
operation, and, after it is executed on the server side, returns the finish message:
// Main.cs
static Random delaygenerator = new Random();
static void ThreadRequest()
{
RemObjects.SDK.BinMessage tempMessage = new RemObjects.SDK.BinMessage();
RemObjects.SDK.IpHttpClientChannel tempChannel = new RemObjects.SDK.IpHttpClientChannel();
tempChannel.TargetUrl = "http://localhost:8099/BIN";
IThreadServerService service = CoThreadServerService.Create(tempMessage, tempChannel);
int delay = delaygenerator.Next(1000, 4000);
MessageBox.Show(service.DoSomethingLong(delay));
}
IMPORTANT: You have to create separate channel, message and proxy instances for every thread that is going to access the server. Neither of those components can be used from several threads simultaneously.