MegaDemo sample (Java)
This is a combined sample demonstrating various features of the Remoting SDK, supported by the Remoting SDK for Java:
- HTTP Channel
- Binary message
- URL schemas
- Simple values and structures transfer
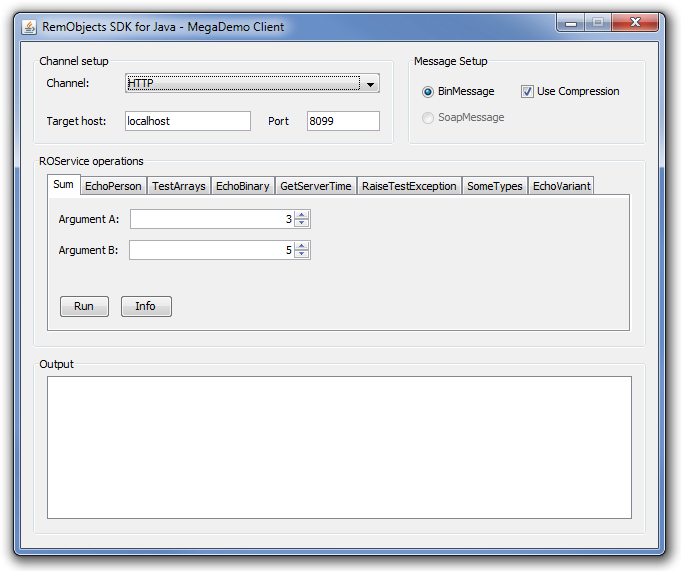
Server Requirements
The MegaDemo Client sample requires the MegaDemo Server either for Delphi or for .NET. Look into the MegaDemo (Delphi) or the MegaDemo (.NET) sample for it.
Getting Started
Build and launch the MegaDemo sample server for the platform you like. Activate HTTP server in the server application. The sample allows you to test the HTTP client channel class with the Binary message class. Data compression can be enabled or disabled for Binary messaging.
To specify the connection settings, the client uses separate controls to set up every parameter. Select the channel type, target host name or IP address, and port.
The default charset for Java is UTF-8. To control the charset for ansi string, the RO SDK provides you to define it yourself:
MegaDemoService.Charset := java.nio.charset.Charset.forName("windows-1251");
MegaDemoService.setCharset(java.nio.charset.Charset.forName("windows-1251"));
NOTE: The default charset for RO SDK AnsiStrings is US-ASCII (according to the canonical name for the java.nio API).
Try to perform different operations with the server. Select a desired tab page, enter the required data into the fields and click the 'Run' button. Click the 'Info' button to show text info about the operation. The process of working is shown in the 'Output' text area. The operations that can be performed on the server are:
- Sum two integer values.
- Send a structure (person type) to the server and back.
- Send an array of different element type (including array of structures) to the server and back.
- Send a binary stream to the server and back.
- Call a parameterless remote function (get server time).
- Handle an exception raised on the server, including exceptions of custom type.
- Send simple types to the server and back.
- Send a Variant to the server and back.
Examine the code
The DemoWindow class is responsible for the sample operations. There are several methods named button<?>MouseClicked. Examine them to see how the sample operations are performed.
Note how the channel and message instances are created with the URI scheme and how to access them:
method DemoWindow.initDemoProxy;
begin
var lProtocol: String := protocolBox.SelectedItem as String;
var lHost: String := hostField.Text as String;
var lPort: Integer := portSpinner.Value as Integer;
var lMessageType: String := '/';
if binaryRbtn.isSelected then
lMessageType := '/bin';
var lScheme := new URI(lProtocol + '://' + lHost + ':' + lPort.toString + lMessageType);
MegaDemoService := new MegaDemoService_Proxy(lScheme);
if useCompession.isSelected then
(MegaDemoService.ProxyMessage as BinMessage).UseCompression := true
else
(MegaDemoService.ProxyMessage as BinMessage).UseCompression := false;
(MegaDemoService.ProxyClientChannel as HttpClientChannel).Timeout := 1000;
end;
public void initDemoProxy() throws URISyntaxException {
String lProtocol = (String) protocolBox.getSelectedItem();
String lHost = (String) hostField.getText();
Integer lPort = (Integer) portSpinner.getValue();
String lMessageType = "/";
if (binaryRbtn.isSelected())
lMessageType = "/bin";
URI lScheme = new URI(lProtocol + "://" + lHost + ":" + lPort.toString() + lMessageType);
MegaDemoService = new MegaDemoService_Proxy(lScheme);
if (useCompession.isSelected())
((BinMessage) MegaDemoService.getProxyMessage()).setUseCompression(true);
else
((BinMessage) MegaDemoService.getProxyMessage()).setUseCompression(false);
((HttpClientChannel) MegaDemoService.getProxyClientChannel()).setTimeout(1000);
}
As you can see, channel and message instances are created implicitly by calling the constructor with the URI parameter. To see all available constructors, please visit the Proxy class page.