MegaDemo sample (Android)
This combined sample demonstrates various features of the Remoting SDK, supported by the Remoting SDK for Java:
- HTTP Channel
- BinMessage|Binary message
- URL Schemes
- Simple values and structures transfer
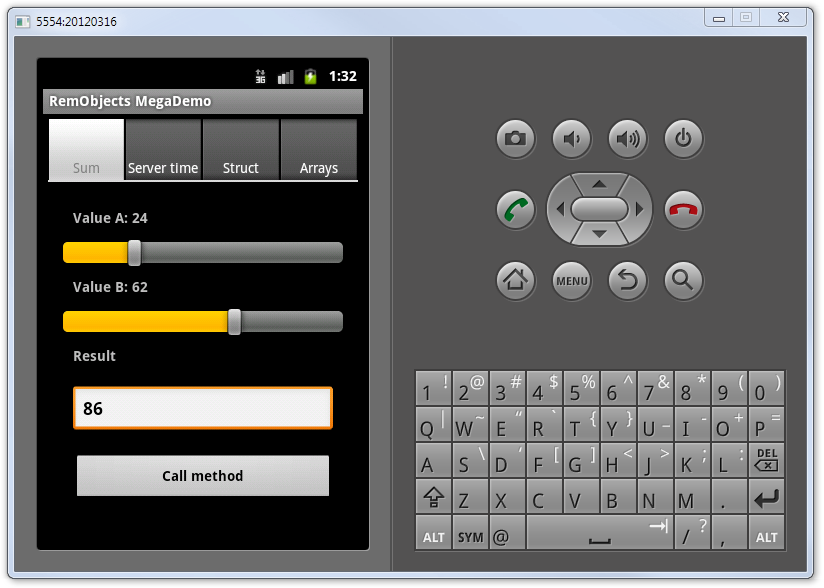
Server Requirements
The MegaDemo Client sample requires the MegaDemo Server either for Delphi or for .NET. Look into the MegaDemo (Delphi) or the MegaDemo (.NET) sample for it.
Getting Started
Build and launch the MegaDemo sample server for the platform you like. Activate the HTTP server in the server application. The sample allows you to test the HTTP client channel class with the Binary message class. Data compression can be enabled or disabled for Binary messaging.
Check the MegaDemoforAndroidActivity
class to modify the following defined URL:
MegaDemoforAndroidActivity.pas
file:
var lScheme := new URI("http://192.168.10.1:8099/bin");
MegaDemoforAndroidActivity.java
file:
URI lScheme = new URI("http://192.168.10.1:8099/bin");
The default charset for Java is UTF-8. To control charset for ansi string the RO SDK provides you to define it your self:
MegaDemoService.Charset := java.nio.charset.Charset.forName("windows-1251");
MegaDemoService.setCharset(java.nio.charset.Charset.forName("windows-1251"));
NOTE: The default charset for RO SDK AnsiStrings is US-ASCII (according to the canonical name for the java.nio API).
Try to perform different operations with the server. Select a desired tab page, enter the required data into the fields and click the Call method
button. The process of working is shown in the Result
text area or Android Toast. The operations that can be performed on the server are:
- Sum two integer values.
- Send a structure (Person type) to the server and back.
- Send an array of different element type (including array of structures) to the server and back.
- Call a parameterless remote function (get server time).
Examine the code
Each operation has its own Activity. There are several Activities named
Pay attention on how the channel and message instances are created with the URI scheme and how to access them:
method MegaDemoforAndroidActivity.initialize;
begin
try
var lScheme := new URI("http://192.168.10.1:8099/bin");
fService := new MegaDemoService_Proxy(lScheme);
(fService.ProxyMessage as BinMessage).UseCompression := true;
(fService.ProxyClientChannel as HttpClientChannel).Timeout := 1000;
except
on E:URISyntaxException do
Log.e("MegaDemoforAndroidActivity", "URISyntaxException: " + E.Message);
end;
end;
private void initialize() {
try {
URI lScheme = new URI("http://192.168.10.1:8099/bin");
aService = new MegaDemoService_Proxy(lScheme);
((BinMessage)aService.getProxyMessage()).setUseCompression(true);
((HttpClientChannel)aService.getProxyClientChannel()).setTimeout(1000);
} catch (URISyntaxException e) {
Log.e("MegaDemoforAndroidActivity", "URISyntaxException: " + e.getMessage());
}
}
As you can see, channel and message instances are created implicitly by calling the constructor with the URI parameter. To see all available constructors, please visit thr Proxy class page.