Sample Server - Bootstrapper
Create a new Windows Forms application named ChatServer.
Remove the unnecessary references and form definitions. Also remove all unnecessary code from the Program.cs file:
using System;
namespace ChatServer
{
static class Program
{
[STAThread]
static void Main()
{
}
}
}
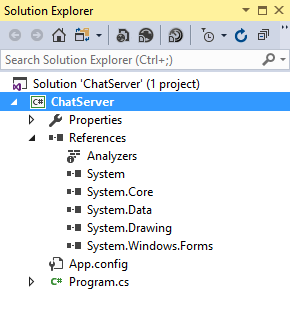
The project is now empty and we can move to the actual server app development.
The first step is to add references to the Remoting SDK assemblies. Assemblies we need to reference are: RemObjects.SDK, RemObjects.SDK.Server.
The next step is to define the network connectivity infrastructure, add the Windows Service support, server console mode, etc. While this might sound scary, the code itself is not:
using System;
using RemObjects.SDK.Server;
namespace ChatServer
{
static class Program
{
[STAThread]
static void Main(string[] args)
{
var server = new ApplicationServer("ChatServer");
server.Run(args);
}
}
}
Also license information should be provided, otherwise the application won't be able to start. Add a new text file named licenses.licx to the project, set its build action to EmbeddedResource. Set the file contents to:
RemObjects.SDK.Server.IpTcpServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.IpSuperHttpServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.IpHttpServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.NamedPipeServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.LocalServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.HttpSysServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.HttpSysSuperHttpServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.SuperTcpServerChannel, RemObjects.SDK.Server
RemObjects.SDK.Server.EventSinkManager, RemObjects.SDK.Server
RemObjects.SDK.Server.MemoryMessageQueueManager, RemObjects.SDK.Server
RemObjects.SDK.Server.MemorySessionManager, RemObjects.SDK.Server
RemObjects.SDK.Server.OlympiaMessageQueueManager, RemObjects.SDK.Server
RemObjects.SDK.Server.OlympiaServerSessionManager, RemObjects.SDK.Server
This will ensure that the compiled assembly will contain all the necessary data to validate licensing at runtime. This file will provide the information required by the .NET Licensing infrastructure to successfully perform its actions on compiling and embedding the licenses.
Now the application can be started. Open the URL http://localhost:8099/ in browser. If everything was done right it will display the server info page:
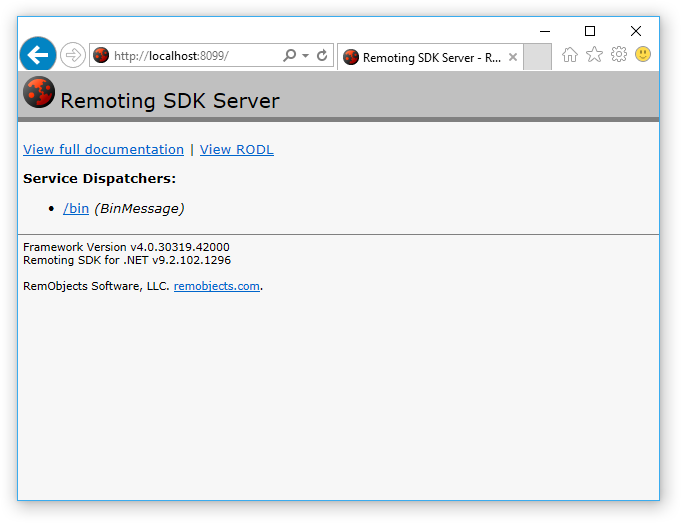
The bootstrapper class used provides a lot of features including:
- Processing command line parameters to run the server in command-line, GUI and Windows Service/Dæmon mode
- Setting up the infrastructure to register, unregister or launch the server as Windows Service
- Providing a default window, in GUI mode
- Setting up the server channel and messages (defaults, or as configured in .exe.config)
- Setting up TLS
- Optionally making sure only a single instance of the server is active
- Providing events for exception handling, as well as startup and shutdown