Sample Client - Receiving data from the server
Open the ChatClient project.
At first it is necessary to update the server interface proxies as it was updated with the Chat events.
This is the point where the .remoteRODL file is used to simplify the update process.
Right-click the ChatServer.remoteRODL file in the Solution Explorer and select the menu item 'Update Interface File(s)'. Already familiar dialog window will appear:
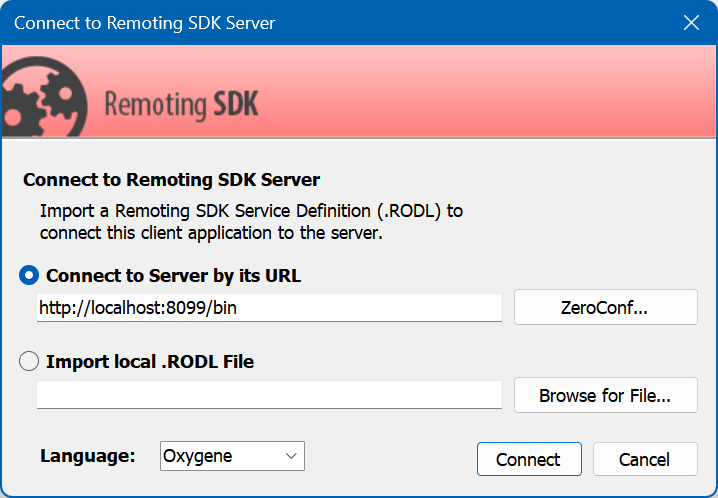
The difference from the first time, now it will show not the default server URI, but the one stored in the .remoteRODL file (still for this tutorial they are the same).
Press the Import button and two more definitions will be added to the ChatServer_Intf.cs file: the IChatEvents interface and the ChatEvents_EventSinkInvoker class.
Now open the MainForm code and add there:
public partial class MainForm : Form, IChatEvents
{
private delegate void GuiUpdate();
void IChatEvents.MessageReceived(string sender, string message)
{
this.Invoke(new GuiUpdate(() => this.ChatLog.Items.Add(string.Format(@"{0}: {1}", sender, message))));
}
<...>
}
This code defines a chat events listener and provides a simple implementation for the event handler. Take a note that event handlers are invoked in a separate non-GUI thread, so the Invoke method call is used to update GUI.
The next step is to define the event receiver that will listen to the data sent to the server and invoke corresponding event handler methods and to register the MainForm instance as event listener.
Add a field definition to the code:
private EventReceiver _eventReceiver;
and add its initialization to the MainForm_Load event handler:
this._eventReceiver = new EventReceiver();
this._eventReceiver.Channel = this._clientChannel;
this._eventReceiver.Message = this._message;
The last step is to register the MainForm instance as event handler during the log in process and to unregister it during the log out process:
private async void LoginButton_Click(object sender, EventArgs e)
{
...
if (loginResult)
{
this._eventReceiver.RegisterEventHandler(this);
}
...
}
private async void LogoutButton_Click(object sender, EventArgs e)
{
...
this._eventReceiver.UnregisterEventHandler(this);
...
}
Start several instances of the Chat Client application and try them in action:
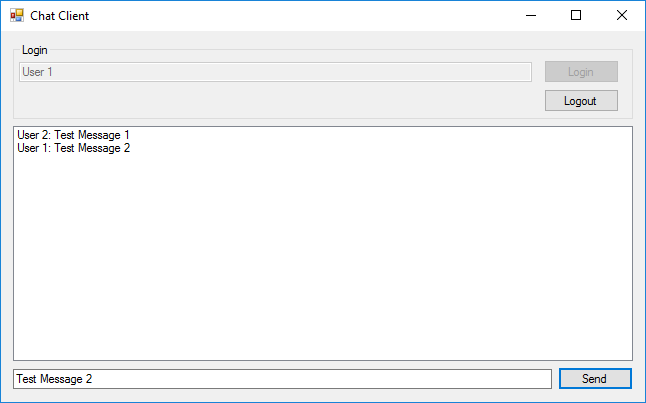
The complete ChatClient project source code can be downloaded here.
At this point the simple tutorial Chat project (client + server) can be considered as complete. It provides a client to server and server to client data flow, user authentication and is based on the Code-First approach.