Connecting to a Remoting SDK Server
Remoting SDK for Cocoa comes with the Service Importer tool that makes it really easy to connect your Cocoa apps to a Remoting SDK server, essentially turning them into a client app.
Open Service Importer, and either specify the URL of your remote server (complete with port and path to RODL, so commonly that will end in :8099/bin
) or alternatively browse for a local .RODL file on disk:
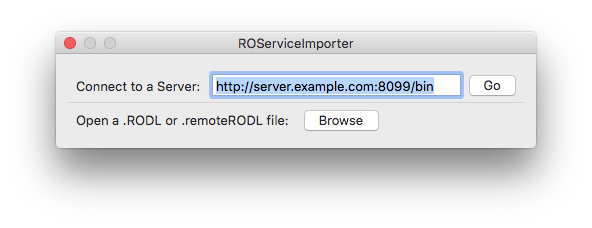
This will open a new document window, import the services and types from the RODL or the remote server, and show the resulting code.
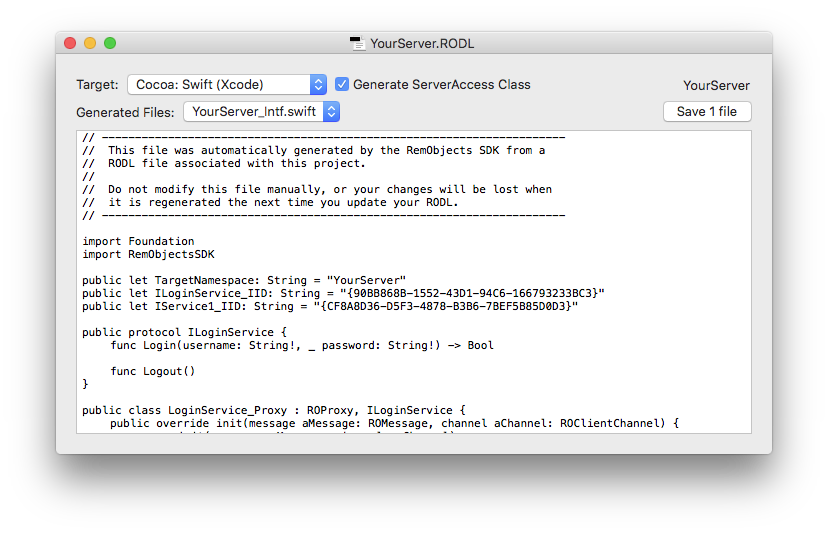
Using the first popup button, you can choose the target platform and language you want to generate code for. Pick either "Swift (Xcode)" or "Objective-C (Xcode)", which are the first two items (Service Importer also supports generating code for the other platforms, and for other languages on the Cocoa platform).
The second popup lets you preview the different files, if you chose a language where multiple files would be generated for the import (such as the .h
and .m
files for Objective-C).
Adding the Files to your Project
Click the "Save files button to generate the code on disk. Browse for your project's location, have the files saved into the same folder, and then simply drag them from Finder into Xcode to add them to your project.
Note that the _ServerAccess file, discussed below, will only be saved if it does not already exist. All other Interface files will be overwritten during save.
If this is your first use of Remoting SDK in your project, you will also want drag a reference to RemObjectsSDK.framework
(located in the folder where you installed Remoting SDK to your project, as well.
Make sure to pick the right version for your platform (i.e. Mac, iOS, tvOS or watchOS). Each version (except for Mac) of the framework is a fat binary that includes all device and simulator architectures for the respective platform.
The Files
Let's look at the generated files, of which there are three:
- a
.remoteRODL
file - an
_Intf
file (or file pair, for Objective-C) - a
_ServerAccess
file (or file pair)
The YourServer.remoteRODL File is a small XML file that serves as the link between your project and the remote server, for this conversion and for future updates. Essentially, it just contains the URL of your server (or of the local .RODL file, if you selected one), and is provided for easy access to working with the server from the IDE. For example, you can update your code to changes in the server via this file, as explained in the Working with .remoteRODL Files topic.
The YourServer_Intf source file contains code generated by Remoting SDK to let you interact with the server. This includes interfaces/protocols for all the Remote Services exposed by your server, as well as any helper types these services use, such as custom enums or structs. You should not touch the code in this file, as it will be re-generated (thus losing any changes you make to it) when you update it to changes on the server. You can read more about _Intf
files here.
Finally, the YourServer_ServerAccess source file contains a small helper class that provides a convenient starting point for encapsulating the access to your server from within your client app. Once created, this file becomes "yours", and you will most likely expand it to expose functionality more specific to your concrete server.
The ServerAccess class is merely a suggestion and an assistance to get you started, you can feel free to simply remove the file from your project if you want to structure your server access differently within the client app.
The ServerAccess
Class
Let's have a closer look at what's inside the ServerAccess class.
This class will differ sightly between the platforms, in order to best fit in with the design patterns and paradigms that make sense on .NET vs. Cocoa vs. Java vs. Delphi. But the principle is the same: It's a singleton class with a single instance, accessible via a static Instance
or sharedInstance
property. It encapsulates your server URL and all the Remoting SDK components needed for the communication, such as the Client Channel and Message instances.
For each service exposed by your remote server, ServerAccess
exposes a public property that gives you easy access to a ready-to-use Proxy class for that service. So all you need to do to make remote calls is to access those properties and call your service methods on them.
If your server uses Authentication via a LoginService
, ServerAccess
will already have that hooked up, and include a stub where you can implement your actual login logic and connect it to any login UI, where needed.
Of course it is up to you to decide which aspects of the pre-generated ServerAccess
fit your application's design and which need changing. For example, you might not want to expose all service proxies publicly, but instead encapsulate access to certain services with bespoke wrapper methods you add to ServerAccess
yourself.