AsyncEx sample (Delphi)
Overview
This sample demonstrates how to call methods on a Remoting SDK server asynchronously using _AsyncEx
interface. This means that the main application thread is not blocked while waiting for the method execution results. This may be especially useful in cases where the operation takes a significant amount of time, so you may submit a request and defer receiving the result for later without creating background threads.
In this sample a very simple calculation is performed, but there is a ten seconds delay in the method so it is possible to query the server before the calculation is completed.
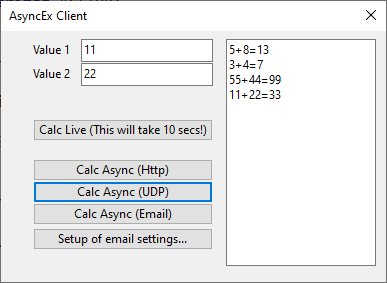
Getting Started
- Compile and launch the server.
- Compile and run the client.
- Click on the
Calc Live
button to verify that the connection works. Notice that the application, its window and all controls in it are frozen until the result is returned. - Try different values via the same or different channels. Results will be received automatically after ten seconds have passed. Notice that the application is not frozen, unlike after clicking the
Calc Live
button.
Examine the code
- Look how the async request to the server is initiated by calling the
beginMethodName
procedure:
procedure TAsyncClientMainForm.CalcAsyncHttpButtonClick(Sender: TObject);
begin
CoAsyncService_AsyncEx.Create(ROBINMessage1, ROWinInetHTTPChannel1).BeginSum(StrToInt(ed_Value1.Text), StrToInt(ed_Value2.Text), Callback, CreateObject);
end;
CreateObject
method just creates string like8+13
and puts it to object for later usage inCallback
method
function TAsyncClientMainForm.CreateObject: TObject;
var
o: TMyObject;
begin
o := TMyObject.Create;
o.Mes := ed_Value1.Text+'+'+ed_Value2.Text;
Result := o;
end;
- Notice how to check if the result of the async call is obtained in
Callback
method:
procedure TAsyncClientMainForm.Callback(const aRequest: IROAsyncRequest);
var
r: string;
begin
r := IntTostr(CoAsyncService_AsyncEx.Create(aRequest.Message, aRequest.Channel).EndSum(aRequest));
Memo1.Lines.Add(TMyObject(aRequest.UserData).Mes+'='+r);
TMyObject(aRequest.UserData).Free;
end;