Arrays sample (Delphi)
Overview
This sample demonstrates how to use Arrays and Structs for representing database tables and a master/detail relationship.
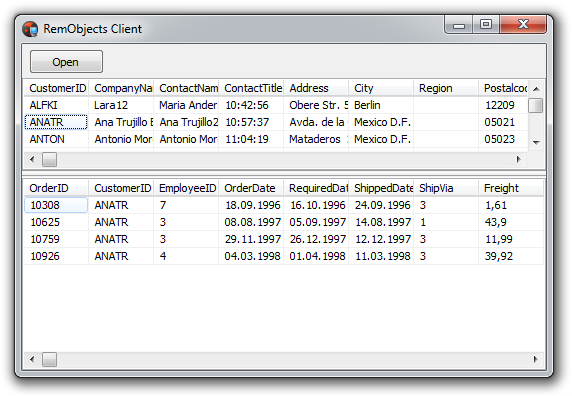
Prerequisites
The sample works with the Northwind database, which has to be available on the localhost server with Windows credentials. In order to adjust the DB connection settings, select the ArraysServerMain form, double-click the TADOConnection component and build the connection string:
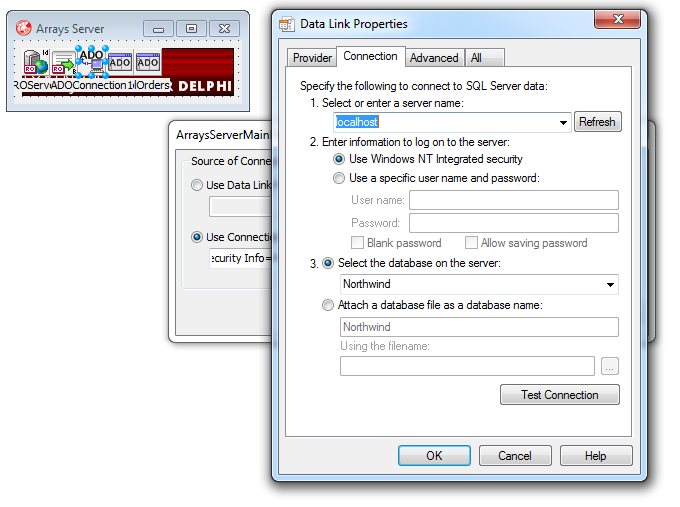
Getting Started
- Compile and launch the server.
- Compile and run the client.
- Click the 'Open' button and both master and detail table views will be filled with data.
- Select different rows in the master table view and see how the data changes in the detail table view.
Examine the Code
The GetTables
method returns a structure containing two arrays:
function TArraysService.GetTables: Tables;
begin
Result := Tables.Create;
// Customers
ArraysServerMainForm.tblCustomers.Close;
ArraysServerMainForm.tblCustomers.Open;
ArraysServerMainForm.tblCustomers.First;
while not ArraysServerMainForm.tblCustomers.Eof do begin
with Result.aCustomers.Add do begin
CustomerID := ArraysServerMainForm.tblCustomers.FieldByName('CustomerID').AsString;
CompanyName := ArraysServerMainForm.tblCustomers.FieldByName('CompanyName').AsString;
// ... and other fields used the same way
end;
ArraysServerMainForm.tblCustomers.Next;
end;
ArraysServerMainForm.tblCustomers.Close;
// orders
// similar to Customers
...
end;
These arrays contain data from the Customers
and Orders
tables and consist of structs matching the table structures:
Customers = class(TROComplexType)
...
published
property CustomerID: UnicodeString read fCustomerID write fCustomerID;
property CompanyName: UnicodeString read fCompanyName write fCompanyName;
// ... similar properties for other fields
end;
...
{ CustomersArray }
CustomersArray_Customers = array of Customers;
CustomersArray = class(TROArray)
private
fCount: Integer;
fItems: CustomersArray_Customers;
...
end;
...
Tables = class(TROComplexType)
...
published
property aCustomers: CustomersArray read GetaCustomers write faCustomers;
property aOrders: OrdersArray read GetaOrders write faOrders;
end;