COM sample (Delphi)
Overview
This sample shows how to call an existing RO server using COM. This allows you to use virtually any programming language and environment on Windows for writing Remoting SDK clients.
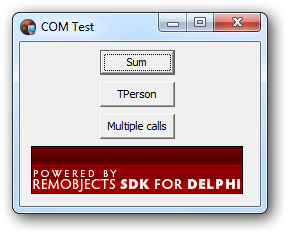
Special Requirements
This sample requires the RO COM automation server to be registered properly. Normally, this is done by the RO installer, but if you need to launch the sample from another computer, you have to do the following:
- Locate the
ROCOM.dll
file in the\RemObjects SDK (Common)\Bin
directory and copy it to the target computer. - On the target computer, use the command line to execute the command from the direcory where
ROCOM.dll
was copied to: .regsvr32 ROCOM.dll
- The command above must be executed as administrator in UAC enabled environments.
This sample has no dedicated server part, it uses the MegaDemo Sample (Delphi) server. For your convenience, the reference to the server project is included into the sample project group.
Getting Started
- Build or compile both projects.
- Launch the MegaDemo Sample (Delphi) server (via the menu option: Tools | RemObjects SDK | Launch Server Executable) and activate the HTTP server.
- Ensure that
COMClient
is the selected project and run it. - Check that the client buttons work as expected.
Examine the Code
- See the simple code needed to invoke the methods in
COM_Main.pas
, the typical code is shown:
procedure TCOM_MainForm.MultipleCallButtonClick(Sender: TObject);
var
roserver: IROCOMServer;
newservice: OleVariant;
person: OleVariant;
begin
roserver := CoROCOMServer.Create;
roserver.MessageType := 'BinMessage';
roserver.ChannelType := 'HTTP';
roserver.SetChannelProperty('TargetURL', 'http://localhost:8099/bin');
person := roserver.CreateComplexType('TPerson');
person.FirstName := 'Peter';
person.LastName := 'Baker';
ShowMessage(person.FirstName + ' ' + person.LastName);
newservice := roserver.CreateService('MegaDemoService');
ShowMessage('The result is ' + IntToStr(newservice.Sum(101, 202)));
ShowMessage('The time on the server is ' + DateTimeToStr(newservice.GetServerTime));
end;
-
The sample also contains several files to show how to call the server from other COM-enabled programming languages and environments:
ROASPDemo.asp
for Active Server Pages sampleExcelDemo.xls
for Visual Basic for Applications sample in the Excel worksheetTest.vbs
for Visual Basic Script sample